Welcome
Hey! I'm Santhosh Nagaraj (yolossn). From dismantling toys as a curious kid to debugging distributed systems today, I've always been driven by the desire to understand how things work under the hood. As a software engineer, I specialize in backend development and cloud-native technologies. While my heart lies in backend architecture, I'm comfortable working across the full stack and love diving into new technologies. When I'm not breaking and rebuilding systems, you'll find me contributing to open-source projects and sharing my learning adventures through writing.
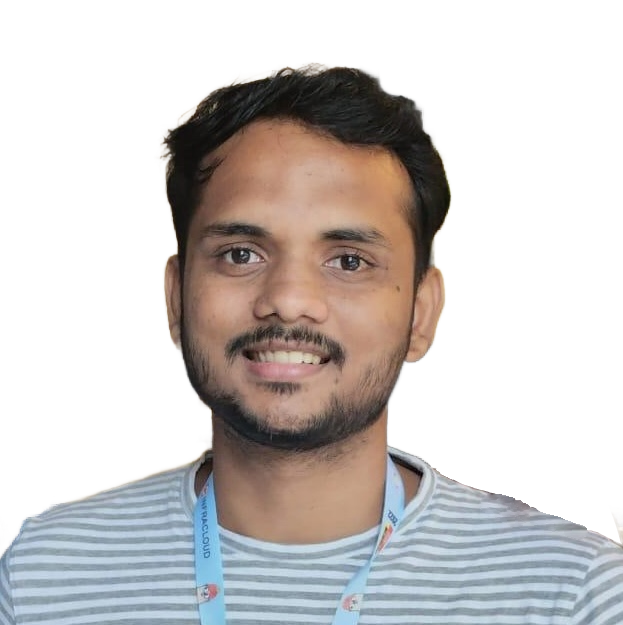
About
Your Time
My Time
Now
Building a browser-based tool that adds TikTok-style captions to videos using Whisper and FFmpeg.
Resume/CV
"Man's search for meaning is the single most important thing in life."